Sort a Python dictionary by value (Python Dictionary Comprehension)
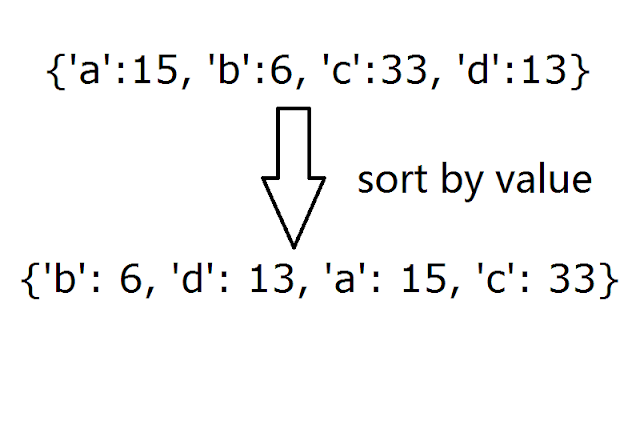
In python, dictionary objects are orderless, there is no way you could sort a dictionary in place like you could do to a list. But you could get a representation of a dictionary, and use an ordered data type to represent sorted values, such as a list of tuples. For instance, d = {'a':15, 'b':6, 'c':33, 'd':13} sorted_d = sorted(d.items(), key=lambda item:item[1]) dict(sorted_d) {'b': 6, 'd': 13, 'a': 15, 'c': 33} or import operator sorted_d = sorted(d.items, key=operator.itemgetter(1)) dict(sorted_d) {'b': 6, 'd': 13, 'a': 15, 'c': 33} In Python 3.6+, we could use dictionary comprehension: {k:v for k,v in sorted(x.items(), key=lambda item:item[1])} {'b': 6, 'd': 13, 'a': 15, 'c': 33}