How to list all files in a directory in Python
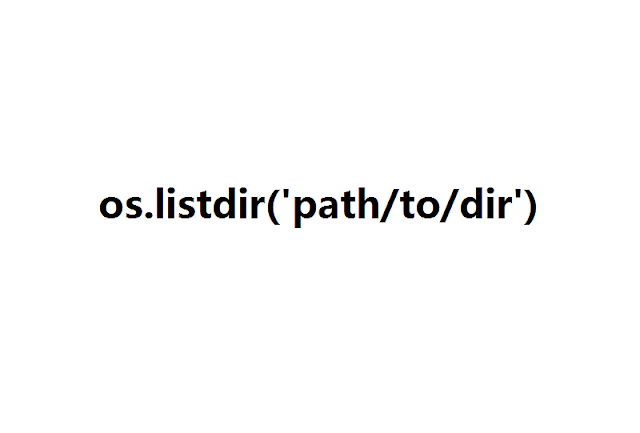
In python, os.listdir() will list everything(files and directories) that is in a directory. >>> import os >>> os.listdir() ['DLLs', 'Doc', 'include', 'Lib', 'libs', 'LICENSE.txt', 'NEWS.txt', 'python.exe', 'python3.dll', 'python38.dll', 'pythonw.exe', 'Scripts', 'tcl', 'Tools', 'vcruntime140.dll'] If you just want files only, you could filter the list like this, >>> from os.path import isfile, join >>> files_only = [file for file in os.listdir() if isfile(join(os.getcwd(),file))] >>> files_only ['LICENSE.txt', 'NEWS.txt', 'python.exe', 'python3.dll', 'python38.dll', 'pythonw.exe', 'vcruntime140.dll'] And also you could use os.walk() , >>> (_,_,files) = next(os.walk(os.getcwd())) >>> files ['LICENSE.txt', 'NEWS.txt', 'python.exe...