What is the difference between list method extend() and operators + or += in Python
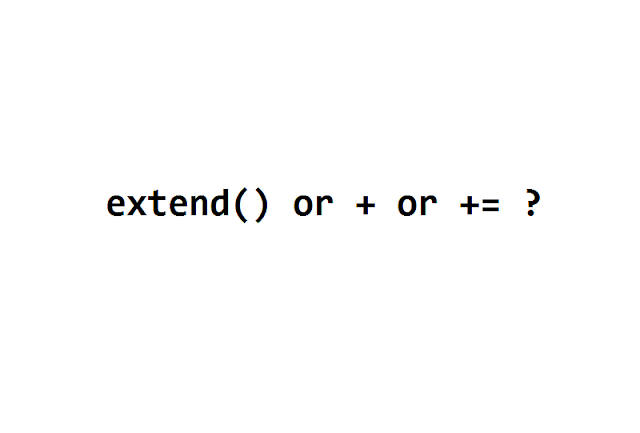
In Python, list support + and += operators. They could do the same thing as the method extend(). extend(): iterate through the iterable object and append each element from the iterable to the end of the list to be extended. Its parameter could be any iterable. >>> a = ['a','b','c'] >>> b = ['x','y','z'] >>> a.extend(b) >>> a ['a', 'b', 'c', 'x', 'y', 'z'] + operator: instead of extending the list in place, it creates a new list leaving original list untouched; it is not as effcient as two others. >>> a = ['a','b','c'] >>> b = ['x','y','z'] >>> c = a + b >>> c ['a', 'b', 'c', 'x', 'y', 'z'] += operator: it extends the list in place which is the same as the extend() method. >>> a = ['a','b','c...